Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- append()
- Machine Learning
- hierarchical_clustering
- len()
- del
- matplotlib
- Python
- sklearn
- 최댓값
- Dictionary
- list
- 반복문
- 덴드로그램
- 분류 결과표
- DataFrame
- count()
- 최솟값
- dendrogram
- numpy
- data
- insert()
- IN
- string
- pandas
- nan
- elbow method
- analizer
- function
- wcss
- DataAccess
Archives
- Today
- Total
개발공부
[Python] Matplotlib Plot(플롯), Bar(바), Pie(파이) Chart 본문
import matplotlib.pyplot as plt
가장 기본적인 Plot
x 좌표의 데이터와, y좌표의 데이터를 가지고 만들수 있는 선형 그래프다.
>>> x = np.arange(0, 9+1)
>>> x
array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
>>> y = np.arange(0, 9+1)
>>> y
array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
plt.plot(x, y)
plt.savefig('test1.jpg')
plt.show()
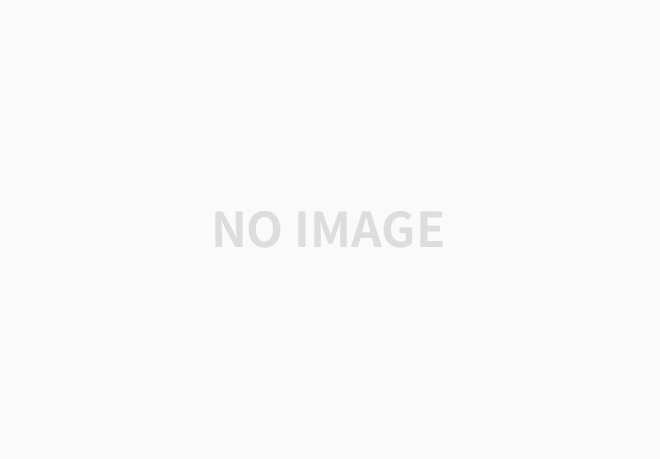
Bar Chart (ceaborn)
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sb
아래는 포켓몬 세대와 종에 대한 정보가 담겨있는 데이터 프레임이다.
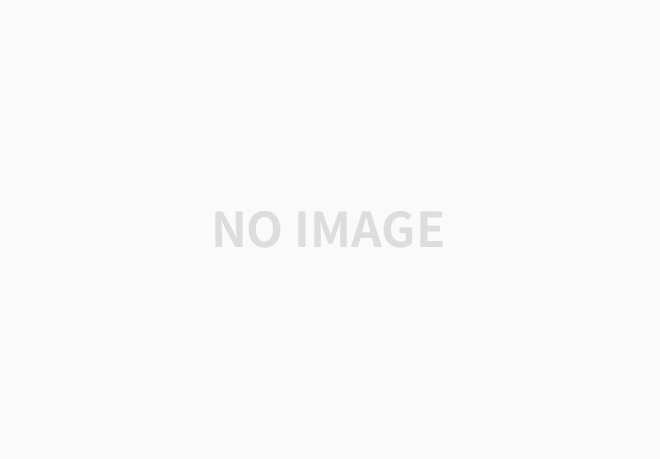
generation_id 별로 각각 몇개씩의 데이터가 있는지를 나타내고 싶을 때
일단 generation_id가 카테고리컬 데이터인지를 확인해보자.
>>> df.shape
(807, 14)
>>> df['generation_id'].nunique()
7
>>> df['generation_id'].unique()
array([1, 2, 3, 4, 5, 6, 7], dtype=int64)
위에보면 df는 807의 행으로 되어 있는데 generation_id는 1부터 7까지로 df이 채워져있으므로
카테고리컬 데이터라고 볼 수 있다.
이제 generation_id별로 각각 몇개씩의 데이터가 있는지는 아래와 같이 찾을 수 있다.
>>> df['generation_id'].value_counts()
5 156
1 151
3 135
4 107
2 100
7 86
6 72
Name: generation_id, dtype: int64
이를 바 차트로 나타내면 아래와 같이 쓸 수 있다.
sb.countplot(data = df, x = 'generation_id')
plt.show() # sb로 썼어라도 plt.show()로 깔끔하게 출력가능
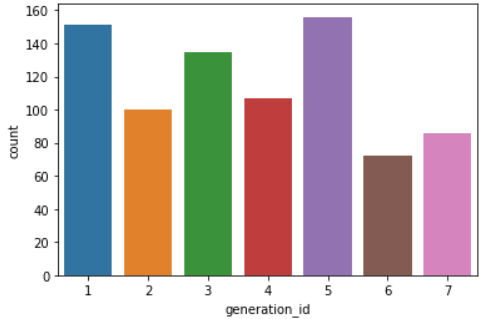
색 바꾸기
seaborn 은 기본적으로 컬러파레트를 내장하고 있다. 이 중 우리가 원하는 색의 인덱스를 찾아 이용할 수 있다.
sb.color_palette()

base_color = sb.color_palette()[2]
sb.countplot(data = df, x = 'generation_id', color = base_color, )
plt.show()
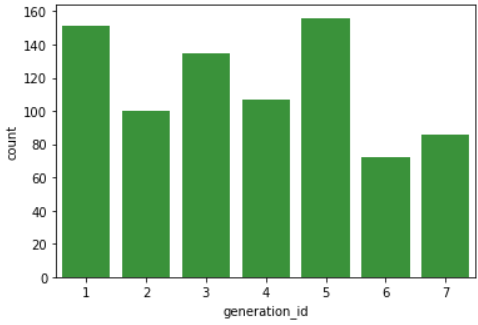
데이터 정렬하기
데이터프레임을 정렬한 것을 저장하고,
이를 사용하여 그래프를 그리면 정렬된 차트를 얻을 수 있다.
>>> my_order = df['generation_id'].value_counts().index
>>> my_order
Int64Index([5, 1, 3, 4, 2, 7, 6], dtype='int64')
>>> sb.countplot(data = df, x = 'generation_id', color = base_color, order = my_order)
>>> plt.show()
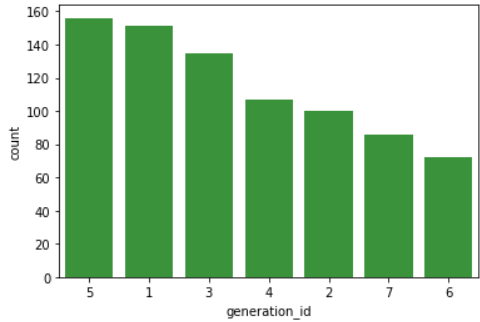
Pie chart
퍼센테이지로 비교해서 보고 싶을때 사용한다.
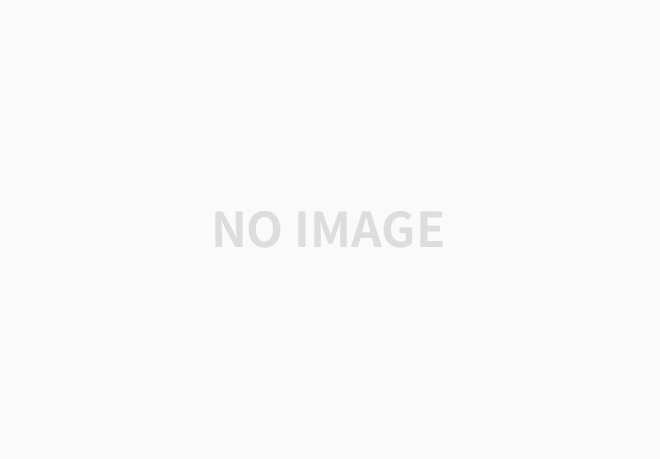
# 각 geeration_id 별로, 파이차트를 그린다.
>>> sorted_data = df['generation_id'].value_counts()
>>> sorted_data
5 156
1 151
3 135
4 107
2 100
7 86
6 72
Name: generation_id, dtype: int64
>>> sorted_data.index
Int64Index([5, 1, 3, 4, 2, 7, 6], dtype='int64')
plt.pie(sorted_data, autopct = '%.1f', labels = sorted_data.index,
startangle = 90, wedgeprops= {'width' : 0.7})
plt.title('Generation Id Pie Chart')
plt.legend() # label별 색상을 표시해준다.
plt.show()
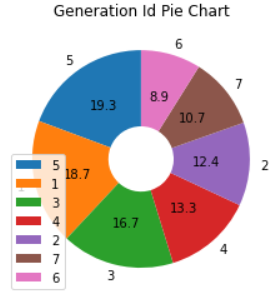
'Python > Matplotlib' 카테고리의 다른 글
[Python] Matplotlib 여러 개의 변수 시각화 방법 (Scatter, seaborn.Regplot, Heat Map), 상관계수 (dataframe.corr()) (0) | 2022.05.04 |
---|---|
[Python] Matplotlib 여러개의 차트를 그릴 수 있는 Subplot (0) | 2022.05.04 |
[Python] Matplotlib Histogram(히스토그램) (0) | 2022.05.03 |